
Types of Servo Motor
DC Servo Motor

AC Servo Motor
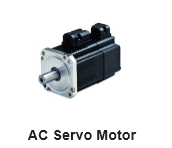
Basic working concept of servo motors

Applications
/* Name : main.c * Purpose : Source code for SERVO MOTOR Interfacing with PIC18F4550. * Author : Gemicates * Date : 2017-06-22 * Website : www.gemicates.org * Revision : None */ #include<xc.h> // Header file for PIC18F4550 #define _XTAL_FREQ 12000000 // 12MHZ void delay_ms(); void delay_us(); unsigned int i; void main() { CMCON = 0x07; // To turn off comparators ADCON1 = 0x0F; // A/D control register TRISC = 0x00; // PORTC as output while(1) { for(i=0;i<49;i++) // rotate to 0 degree { PORTCbits.RC0=1; __delay_us(900); PORTCbits.RC0=0; __delay_us(19100); } __delay_ms(20); // delay for 0.02 sec { for(i=0;i<49;i++) // rotate to 45 degree { PORTCbits.RC0=1; __delay_us(1250); PORTCbits.RC0=0; __delay_us(18750); } __delay_ms(20); // delay for 0.02 sec { for(i=0;i<49;i++) // rotate to 90 degree { PORTCbits.RC0=1; __delay_us(1500); PORTCbits.RC0=0; __delay_us(18500); } __delay_ms(20); // delay for 0.02 sec { for(i=0;i<49;i++) // rotate to 1350 degree { PORTCbits.RC0=1; __delay_us(1750); PORTCbits.RC0=0; __delay_us(18250); } __delay_ms(20); // delay for 0.02 sec { for(i=0;i<49;i++) // rotate to 180 degree { PORTCbits.RC0=1; __delay_us(2000); PORTCbits.RC0=0; __delay_us(18000); } __delay_ms(20); // delay for 0.02 sec } } } } } }